Introduction
Object-oriented programming (OOP) is a paradigm that uses "objects" to design software. An object is a collection of data (attributes) and methods (functions) that act on the data. OOP helps in organizing code and making it more modular, reusable, and easier to manage. Python, being an object-oriented language, is ideal for learning these concepts.
Key Concepts of OOP
- Class: A blueprint for creating objects.
- Object: An instance of a class.
- Attributes: Variables that store data for an object.
- Methods: Functions that define behaviors for an object.
- Inheritance: Mechanism for creating a new class using details of an existing class.
- Encapsulation: Bundling data and methods within a class.
- Polymorphism: Ability to use a method in different ways.
Basic Structure of a Class in Python
Here's an example to illustrate the OOP concepts in Python:
class Vehicle:
def __init__(self, make, model, year):
self.make = make
self.model = model
self.year = year
def display_info(self):
print(f"{self.year} {self.make} {self.model}")
# Inheritance
class Car(Vehicle):
def __init__(self, make, model, year, doors):
super().__init__(make, model, year)
self.doors = doors
def display_info(self):
super().display_info()
print(f"Doors: {self.doors}")
# Creating objects
vehicle1 = Vehicle("Toyota", "Corolla", 2020)
car1 = Car("Honda", "Civic", 2019, 4)
# Using methods
vehicle1.display_info()
car1.display_info()
Explanation
1. Class Definition:
class Vehicle:
This line defines a new class called Vehicle
.
2. Constructor Method (__init__
):
def __init__(self, make, model, year):
self.make = make
self.model = model
self.year = year
The __init__
method initializes the object's attributes.
3. Instance Method:
def display_info(self):
print(f"{self.year} {self.make} {self.model}")
This method prints the vehicle's information.
4. Inheritance:
class Car(Vehicle):
def __init__(self, make, model, year, doors):
super().__init__(make, model, year)
self.doors = doors
The Car
class inherits from the Vehicle
class. The super()
function is used to call the parent class's __init__
method.
5. Overriding Methods:
def display_info(self):
super().display_info()
print(f"Doors: {self.doors}")
The display_info
method is overridden in the Car
class to include additional information.
6. Creating Objects:
vehicle1 = Vehicle("Toyota", "Corolla", 2020)
car1 = Car("Honda", "Civic", 2019, 4)
These lines create instances of the Vehicle
and Car
classes.
7. Using Methods:
vehicle1.display_info()
car1.display_info()
These lines call the display_info
method for each object.
Benefits of OOP
- Modularity: Breaks down complex problems into smaller, manageable pieces.
- Reusability: Classes can be reused across different parts of the program.
- Abstraction: Hides complex implementation details and exposes only necessary parts.
- Encapsulation: Enhances data protection by binding data and methods within one unit.
Conclusion
Object-oriented programming is a powerful way to structure your code, making it more modular and reusable. Python's simplicity makes it an excellent language to learn and implement OOP concepts. By understanding and practicing these basics, you'll be able to write more organized and efficient code.
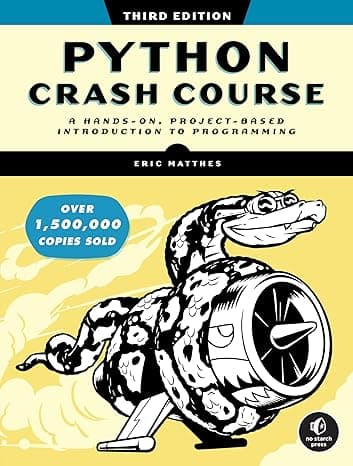