Introduction
Classes are fundamental concepts in computer science and programming, especially in object-oriented programming (OOP). They help in structuring your code, making it more modular, reusable, and easier to manage. This article will guide you through the basics of classes using Python, a popular programming language known for its simplicity and readability.
What is a Class?
A class is a blueprint for creating objects (a particular data structure). Objects are instances of classes. A class encapsulates data for the object and methods to manipulate that data. Think of a class as a template, and an object as an instance of that template.
Basic Structure of a Class in Python
Here’s a simple example to illustrate the concept of a class:
class Animal:
def __init__(self, name, species):
self.name = name
self.species = species
def make_sound(self, sound):
print(f'{self.name} says {sound}')
# Creating an instance of the Animal class
dog = Animal("Buddy", "Dog")
cat = Animal("Whiskers", "Cat")
# Using the make_sound method
dog.make_sound("Woof")
cat.make_sound("Meow")
Explanation
- Class Definition:
class Animal:
This line defines a new class called Animal
.
- Constructor Method (
__init__
):
def __init__(self, name, species):
self.name = name
self.species = species
The __init__
method is a special method called a constructor. It is used to initialize the object's state. The self
parameter refers to the current instance of the class, allowing you to access its attributes and methods.
- Instance Attributes:
self.name = name
self.species = species
These lines initialize the name
and species
attributes for the class.
- Instance Method:
def make_sound(self, sound):
print(f'{self.name} says {sound}')
This method allows the object to perform an action. In this case, it prints the sound made by the animal.
- Creating Objects:
dog = Animal("Buddy", "Dog")
cat = Animal("Whiskers", "Cat")
These lines create two instances (objects) of the Animal
class.
- Using Methods:
dog.make_sound("Woof")
cat.make_sound("Meow")
These lines call the make_sound
method for each object, printing the sound they make.
Benefits of Using Classes
- Modularity: Classes allow you to break down complex problems into smaller, manageable pieces.
- Reusability: Once a class is written, it can be reused across different parts of your program or in different programs.
- Abstraction: Classes help in hiding the complex implementation details and exposing only the necessary parts.
- Encapsulation: By binding data and methods that operate on the data within one unit (class), it enhances data protection and avoids accidental interference.
Conclusion
Understanding classes is crucial for mastering object-oriented programming. By defining classes, you create a blueprint for objects, making your code more organized and modular. Python’s simple syntax makes it an excellent language to learn these concepts. Start with basic examples, practice by creating your own classes, and gradually move to more complex structures to deepen your understanding.
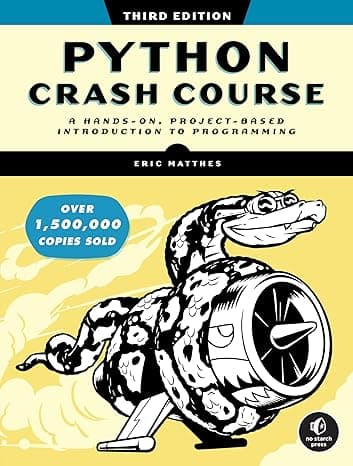