Introduction to LinkedList
In computer science, a LinkedList is a fundamental data structure that consists of a sequence of elements where each element points to the next one in the sequence. Unlike arrays, LinkedLists do not store elements in contiguous memory locations, offering dynamic size adjustments and efficient insertions and deletions. Let's delve into LinkedLists in C# to understand their usage, advantages, and implementation.
Understanding LinkedList in C#
Creating a LinkedList
In C#, LinkedLists are implemented using the LinkedList<T>
class, where <T>
denotes a generic type parameter. This class, found in the System.Collections.Generic
namespace, allows LinkedLists to store elements of any specified data type, offering flexibility and efficient data management capabilities.
// Creating an empty LinkedList of integers
LinkedList<int> linkedList = new LinkedList<int>();
Adding Elements to a LinkedList
Elements can be added to a LinkedList using various methods, such as AddFirst
, AddLast
, and AddAfter
.
// Adding elements to the LinkedList
linkedList.AddLast(10);
linkedList.AddLast(20);
linkedList.AddLast(30);
Removing Elements from a LinkedList
Likewise, elements can be removed using methods like Remove
, RemoveFirst
, and RemoveLast
.
// Removing elements from the LinkedList
linkedList.Remove(20); // Removes the element with value 20
Iterating Through a LinkedList
LinkedLists can be traversed using foreach
loops or using the LinkedListNode<T>
type to navigate between nodes.
// Iterating through the LinkedList
foreach (int value in linkedList)
{
Console.WriteLine(value);
}
// Using LinkedListNode for more control
LinkedListNode<int> currentNode = linkedList.First;
while (currentNode != null)
{
Console.WriteLine(currentNode.Value);
currentNode = currentNode.Next;
}
Advantages of LinkedList
- Dynamic Size: LinkedLists can grow or shrink dynamically.
- Efficient Insertions and Deletions: Operations are efficient as they involve adjusting pointers rather than shifting elements.
- Versatility: Suitable for implementing queues, stacks, and other dynamic data structures.
Practical Applications of LinkedList
LinkedLists are commonly used in scenarios where frequent insertions and deletions are required, such as:
- Implementing queues and stacks.
- Managing memory efficiently in systems programming.
- Maintaining a sorted collection of data.
Best Practices for Using LinkedList
- Node Management: Properly manage LinkedList nodes to avoid memory leaks.
- Performance Considerations: Be mindful of traversal and access patterns for optimal performance.
- Usage Scenarios: Evaluate if LinkedLists are the best fit based on operations and requirements.
Conclusion
LinkedLists in C# provide a flexible and efficient way to manage collections of data, offering advantages over traditional arrays in certain scenarios. By mastering LinkedLists and understanding their implementation details, developers can leverage them effectively to build robust and scalable applications. This blog post has equipped you with a solid foundation in LinkedLists, from basic operations to practical applications.
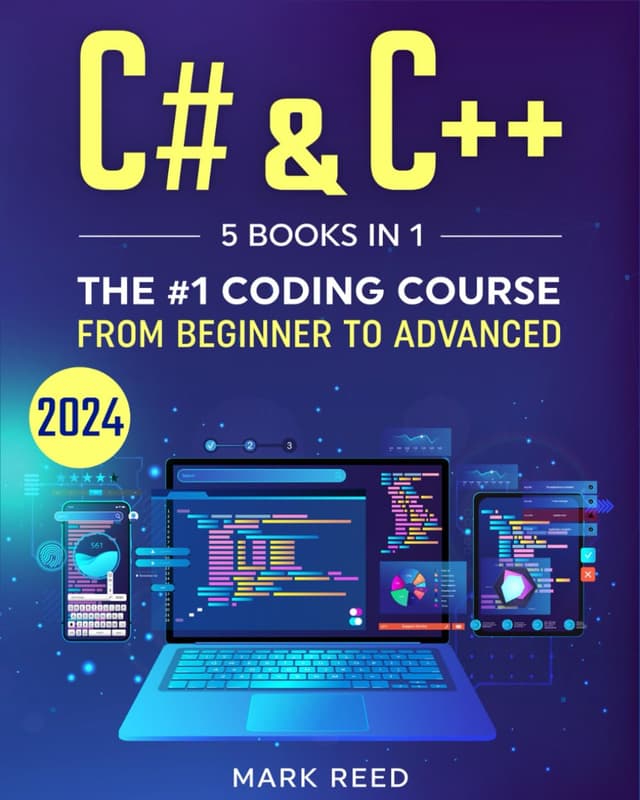