Introduction
In the world of computer science, efficient data storage and retrieval are paramount. One of the key tools in achieving this efficiency is the hash table, a powerful data structure that allows for fast access to stored data. In this article, we'll delve into what hash tables are, how they work, demonstrate their implementation in C#, and explore real-world applications where they excel.
What is a Hash Table?
A hash table (or hash map) is a data structure that implements an associative array abstract data type, a structure that can map keys to values. It uses a hash function to compute an index into an array of buckets or slots, from which the desired value can be found. This indexing structure allows for rapid lookup, insertions, and deletions.
How Hash Tables Work
The core idea behind a hash table is the hash function. This function takes an input (typically the key of the data to be stored) and computes a numeric value, known as a hash code or hash value. This hash code is then used as an index to store or retrieve the data associated with the key.
Key characteristics of a hash table include:
- Hash Function: Determines how keys are mapped to indices in the array.
- Collision Handling: Occurs when two keys hash to the same index. Techniques like chaining (using linked lists at each array index) or open addressing (finding alternative locations) handle collisions.
- Load Factor: A measure of how full the hash table is, affecting performance and space efficiency.
Implementing a Hash Table in C#
Let's walk through a simple implementation of a hash table in C#, focusing on basic operations: inserting a key-value pair, retrieving a value by key, and handling collisions using chaining.
using System;
using System.Collections.Generic;
public class HashTable<TKey, TValue>
{
private const int Capacity = 100; // Adjust capacity as needed
private LinkedList<(TKey key, TValue value)>[] data;
public HashTable()
{
data = new LinkedList<(TKey, TValue)>[Capacity];
}
private int HashFunction(TKey key)
{
// Example of a basic hash function
return key.GetHashCode() % Capacity;
}
public void Add(TKey key, TValue value)
{
int index = HashFunction(key);
if (data[index] == null)
{
data[index] = new LinkedList<(TKey, TValue)>();
}
data[index].AddLast((key, value));
}
public TValue Get(TKey key)
{
int index = HashFunction(key);
if (data[index] != null)
{
foreach (var item in data[index])
{
if (item.key.Equals(key))
{
return item.value;
}
}
}
throw new KeyNotFoundException("Key not found in hash table.");
}
}
Real-World Use Cases of Hash Tables
Hash tables find extensive use in various real-world applications due to their efficient data retrieval capabilities. Here are some common scenarios where hash tables are employed:
1. Database Indexing:
Hash tables are frequently used in database indexing to speed up data retrieval. Database systems often employ hash tables to store and quickly locate records based on their primary keys or indexed columns. This accelerates search operations and improves overall database performance.
2. Caching Mechanisms:
In web applications and systems requiring fast access to frequently accessed data, hash tables serve as an excellent choice for caching mechanisms. For instance, caching API responses or web page content using hash tables can significantly reduce response times and server load.
3. Symbol Tables in Compilers:
Compilers and interpreters use hash tables as symbol tables to manage identifiers such as variable names, function names, and keywords within a program. This allows quick resolution of identifiers during parsing and semantic analysis phases.
4. Implementing Hash Sets and Hash Maps:
Hash tables are the foundational data structure for implementing hash sets and hash maps (or dictionaries). These data structures are crucial in many programming tasks, including storing unique elements, counting frequencies, and mapping keys to values efficiently.
5. Network Packet Routing:
Network routers and switches often use hash tables to efficiently route packets based on destination IP addresses or other header fields. This ensures that network traffic is handled swiftly, even in complex networks with high data throughput.
6. Password Storage and Authentication:
Hash tables (specifically, hash functions like SHA-256) play a crucial role in securely storing passwords in databases. By storing hashed passwords instead of plaintext, hash tables help protect user credentials from unauthorized access and ensure data security.
Conclusion
Hash tables are indispensable in computer science and software engineering for their ability to provide efficient data storage and retrieval. Whether optimizing database queries, accelerating web applications, or securing sensitive information, understanding and leveraging hash tables is key to developing robust and performant software solutions.
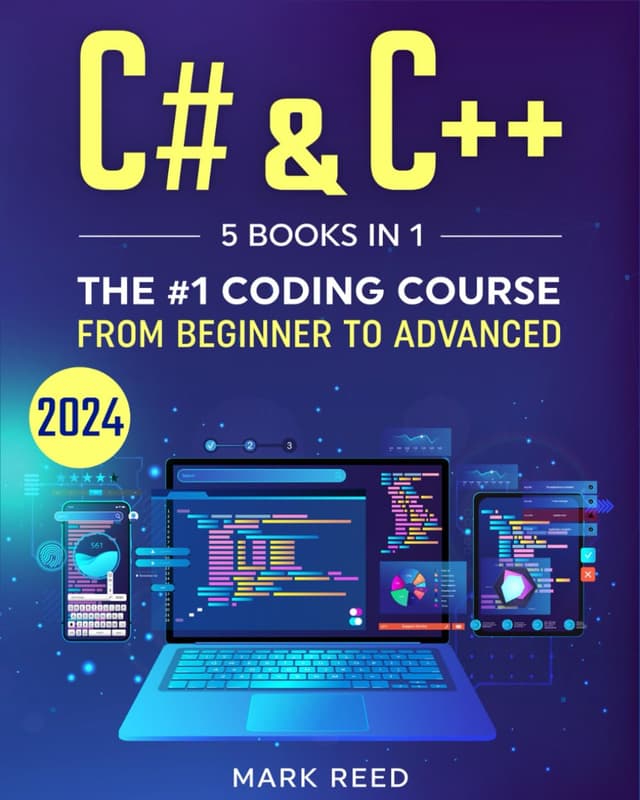