Introduction
Programming involves making decisions, and conditional statements are the cornerstone of decision-making in code. One such powerful control structure is the switch case statement. This article will delve into the switch case statement in C#, exploring its syntax, usage, and practical examples, providing you with a solid understanding of how to implement it effectively in your programs.
Understanding the Switch Case Statement
In computer science, the switch case statement is a powerful control structure that allows you to choose between multiple options based on the value of a variable or expression. It simplifies complex conditional statements involving multiple conditions, evaluates an expression, and executes the corresponding code block based on the matching case. This helps in writing cleaner and more readable code compared to multiple if-else-if statements.
Basic Syntax
Here’s the basic syntax of a switch case statement in C#:
switch (expression)
{
case value1:
// Code to execute if expression equals value1
break;
case value2:
// Code to execute if expression equals value2
break;
// More cases...
default:
// Code to execute if no case matches
break;
}
Example: Simple Switch Case
Let's start with a simple example that demonstrates the use of a switch case statement.
class Program
{
static void Main()
{
int dayOfWeek = 3;
switch (dayOfWeek)
{
case 1:
Console.WriteLine("Monday");
break;
case 2:
Console.WriteLine("Tuesday");
break;
case 3:
Console.WriteLine("Wednesday");
break;
case 4:
Console.WriteLine("Thursday");
break;
case 5:
Console.WriteLine("Friday");
break;
case 6:
Console.WriteLine("Saturday");
break;
case 7:
Console.WriteLine("Sunday");
break;
default:
Console.WriteLine("Invalid day");
break;
}
}
}
In this example, the variable dayOfWeek
is evaluated, and since its value is 3, the program outputs "Wednesday."
Using Enums with Switch Case
Enums (short for enumerations) are a great way to define a set of named constants, and they work well with switch cases.
enum DayOfWeek
{
Monday = 1,
Tuesday,
Wednesday,
Thursday,
Friday,
Saturday,
Sunday
}
class Program
{
static void Main()
{
DayOfWeek today = DayOfWeek.Wednesday;
switch (today)
{
case DayOfWeek.Monday:
Console.WriteLine("It's Monday");
break;
case DayOfWeek.Tuesday:
Console.WriteLine("It's Tuesday");
break;
case DayOfWeek.Wednesday:
Console.WriteLine("It's Wednesday");
break;
case DayOfWeek.Thursday:
Console.WriteLine("It's Thursday");
break;
case DayOfWeek.Friday:
Console.WriteLine("It's Friday");
break;
case DayOfWeek.Saturday:
Console.WriteLine("It's Saturday");
break;
case DayOfWeek.Sunday:
Console.WriteLine("It's Sunday");
break;
default:
Console.WriteLine("Invalid day");
break;
}
}
}
This example uses an enum DayOfWeek
to define the days of the week and a switch case to output the corresponding day.
Practical Application: Simple Calculator
Let’s use the switch case statement to create a simple calculator that performs basic arithmetic operations.
class Program
{
static void Main()
{
Console.WriteLine("Enter first number:");
double num1 = Convert.ToDouble(Console.ReadLine());
Console.WriteLine("Enter second number:");
double num2 = Convert.ToDouble(Console.ReadLine());
Console.WriteLine("Enter operation (+, -, *, /):");
char operation = Console.ReadKey().KeyChar;
Console.WriteLine();
switch (operation)
{
case '+':
Console.WriteLine($"Result: {num1 + num2}");
break;
case '-':
Console.WriteLine($"Result: {num1 - num2}");
break;
case '*':
Console.WriteLine($"Result: {num1 * num2}");
break;
case '/':
if (num2 != 0)
{
Console.WriteLine($"Result: {num1 / num2}");
}
else
{
Console.WriteLine("Cannot divide by zero");
}
break;
default:
Console.WriteLine("Invalid operation");
break;
}
}
}
This program reads two numbers and an operator from the user, then uses a switch case to perform the corresponding arithmetic operation and display the result.
Benefits of Using Switch Case
- Clarity and Readability: Switch case statements are often more readable than multiple if-else-if chains, making the code easier to understand and maintain.
- Efficiency: In some cases, switch statements can be more efficient than if-else chains, especially when dealing with multiple conditions.
- Organized Code: Using switch case statements helps organize code into distinct, manageable blocks based on different values of an expression.
Conclusion
The switch case statement is a versatile and efficient control structure in C# that simplifies complex decision-making processes in your programs. By understanding and using switch cases, you can write cleaner, more readable code that is easier to maintain and debug. Mastering the switch case statement will enhance your ability to create robust and efficient applications.
In summary, the switch case statement is an essential tool in C# programming, providing a structured way to handle multiple conditions and streamline your code.
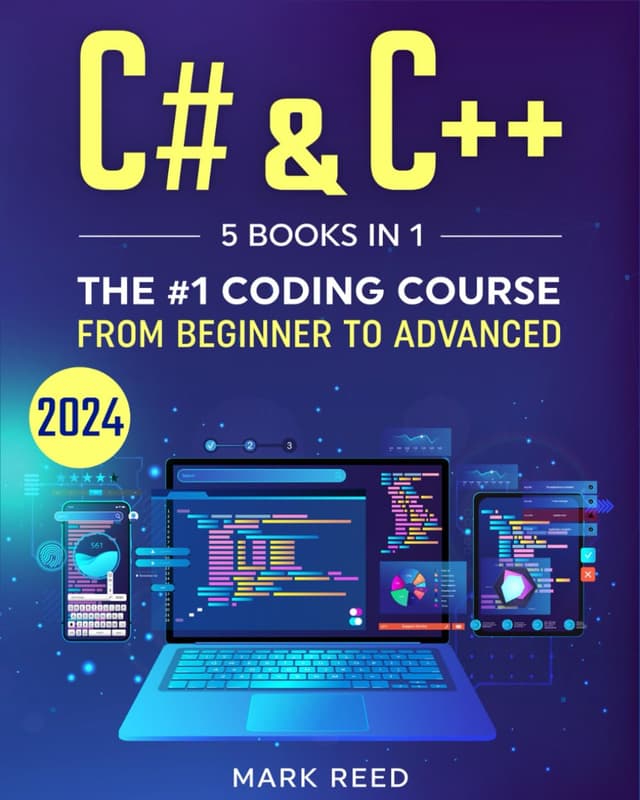