Introduction
In computer science, a stack is a fundamental data structure that operates on a Last In, First Out (LIFO) principle. This means that the last element added to the stack will be the first one to be removed. This article explores the concept of a stack, its operations, and provides practical examples using C# to illustrate its implementation.
What is a Stack?
A stack is a linear data structure that allows operations at only one end, called the top of the stack. The primary operations of a stack are:
- Push: Adding an element to the top of the stack.
- Pop: Removing the top element from the stack.
- Peek: Viewing the top element without removing it.
- IsEmpty: Checking if the stack is empty.
Why Use a Stack?
Stacks are used in various applications such as:
- Function Call Management: Keeping track of function calls and local variables.
- Expression Evaluation: Converting and evaluating expressions in calculators.
- Undo Mechanism: Implementing undo features in text editors and other applications.
Example: Implementing a Stack in C#
Let's create a simple stack class in C# to understand how these operations work:
using System;
using System.Collections.Generic;
public class Stack<T>
{
private List<T> elements = new List<T>();
// Push operation
public void Push(T item)
{
elements.Add(item);
}
// Pop operation
public T Pop()
{
if (IsEmpty())
{
throw new InvalidOperationException("The stack is empty.");
}
T value = elements[elements.Count - 1];
elements.RemoveAt(elements.Count - 1);
return value;
}
// Peek operation
public T Peek()
{
if (IsEmpty())
{
throw new InvalidOperationException("The stack is empty.");
}
return elements[elements.Count - 1];
}
// IsEmpty operation
public bool IsEmpty()
{
return elements.Count == 0;
}
}
class Program
{
static void Main()
{
Stack<int> stack = new Stack<int>();
stack.Push(1);
stack.Push(2);
stack.Push(3);
Console.WriteLine("Top element is: " + stack.Peek()); // Outputs: 3
Console.WriteLine("Popped element is: " + stack.Pop()); // Outputs: 3
Console.WriteLine("Popped element is: " + stack.Pop()); // Outputs: 2
Console.WriteLine("Is stack empty? " + stack.IsEmpty()); // Outputs: False
stack.Pop(); // Pops the last element (1)
Console.WriteLine("Is stack empty? " + stack.IsEmpty()); // Outputs: True
}
}
Explanation
- Push Operation: Adds an element to the top of the stack using
Add
method of theList<T>
class. - Pop Operation: Removes and returns the top element of the stack. It first checks if the stack is empty to avoid underflow.
- Peek Operation: Returns the top element without removing it, again checking if the stack is empty.
- IsEmpty Operation: Checks if the stack has any elements.
Benefits of Using a Stack
- Simple to Implement: Stacks are straightforward to implement using arrays or linked lists.
- Efficient Memory Use: Stacks dynamically adjust their size, making efficient use of memory.
- Useful for Many Algorithms: Many algorithms and applications benefit from the LIFO nature of stacks, making them versatile.
Conclusion
Stacks are a crucial data structure in computer science, essential for managing function calls, evaluating expressions, and implementing undo mechanisms, among other applications. By understanding and implementing stacks in C#, developers can harness the power of this simple yet effective data structure in their projects.
In summary, mastering stacks and their operations lays a strong foundation for tackling more complex data structures and algorithms in computer science.
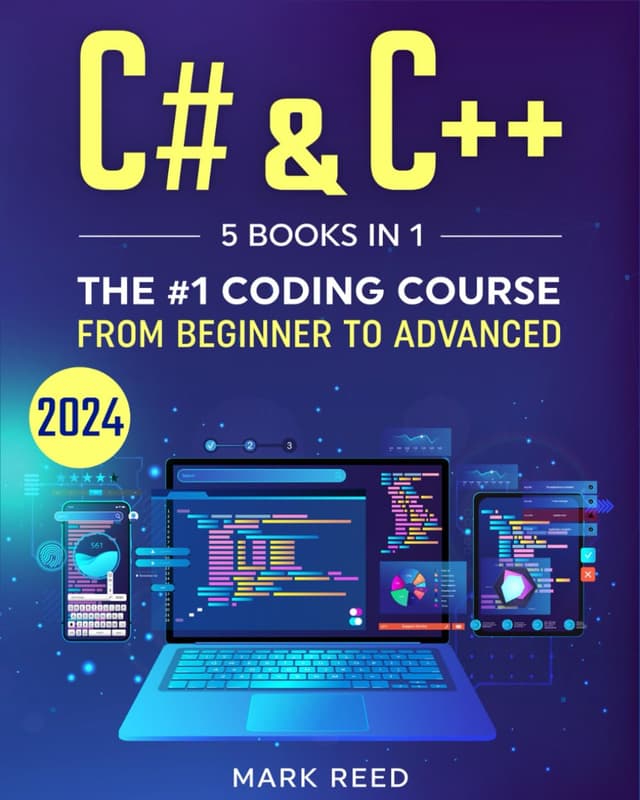