Introduction to Object-Oriented Programming
Object-Oriented Programming (OOP) is a fundamental paradigm in software development that revolves around the concept of objects. Each object is an instance of a class, encapsulating data (attributes or fields) and behaviors (methods or functions). In this blog post, we'll explore the key principles of OOP using C# and provide practical examples to illustrate these concepts.
Key Principles of Object-Oriented Programming
1. Encapsulation
Encapsulation refers to bundling data (attributes) and methods (functions) that operate on the data within a single unit, i.e., a class. It ensures that the internal state of an object is protected from outside interference and manipulation.
Example: Encapsulation in C#
public class Employee
{
private string name;
private int age;
public Employee(string empName, int empAge)
{
name = empName;
age = empAge;
}
public void DisplayDetails()
{
Console.WriteLine($"Name: {name}, Age: {age}");
}
}
2. Inheritance
Inheritance allows one class (derived class or subclass) to inherit the properties and behaviors of another class (base class or superclass). It promotes code reusability and supports the "is-a" relationship between classes.
Example: Inheritance in C#
public class Manager : Employee
{
private string department;
public Manager(string empName, int empAge, string dept)
: base(empName, empAge)
{
department = dept;
}
public void DisplayDetails()
{
base.DisplayDetails();
Console.WriteLine($"Department: {department}");
}
}
3. Polymorphism
Polymorphism allows objects of different classes to be treated as objects of a common superclass. It enables methods to be overridden in derived classes to provide different implementations while still adhering to a common interface.
Example: Polymorphism in C#
public class Shape
{
public virtual void Draw()
{
Console.WriteLine("Drawing a shape");
}
}
public class Circle : Shape
{
public override void Draw()
{
Console.WriteLine("Drawing a circle");
}
}
public class Rectangle : Shape
{
public override void Draw()
{
Console.WriteLine("Drawing a rectangle");
}
}
Applying Object-Oriented Programming in C#
Now, let's combine these principles to create a cohesive example.
Example: Using OOP Principles Together
class Program
{
static void Main(string[] args)
{
// Creating an instance of Employee
Employee emp = new Employee("John Doe", 30);
emp.DisplayDetails();
// Creating an instance of Manager (inherits from Employee)
Manager manager = new Manager("Jane Smith", 40, "IT");
manager.DisplayDetails();
// Using polymorphism
Shape[] shapes = new Shape[] { new Circle(), new Rectangle() };
foreach (Shape shape in shapes)
{
shape.Draw();
}
}
}
Benefits of Object-Oriented Programming
- Modularity: Code is divided into manageable units (classes), promoting easier maintenance and updates.
- Reusability: Classes and their components can be reused in different parts of the application.
- Scalability: Supports building complex systems by modeling real-world entities as objects.
- Encapsulation: Protects data from unauthorized access and modification, enhancing security.
Conclusion
Object-Oriented Programming is a powerful paradigm that enhances code structure, reusability, and maintainability. By leveraging encapsulation, inheritance, and polymorphism, developers can create robust and efficient software solutions. In this blog post, we've explored these concepts through practical examples in C#, demonstrating their application in real-world scenarios.
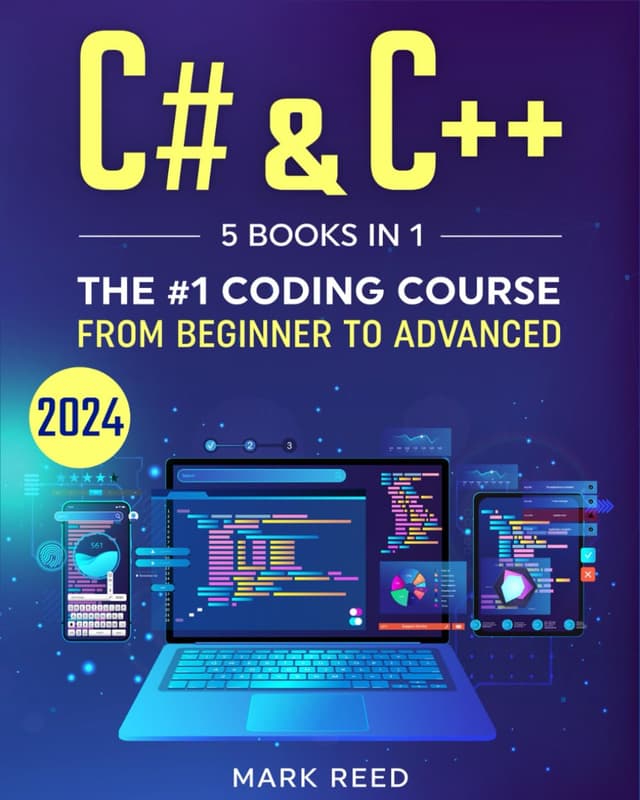