Introduction to Arrays
Arrays are fundamental data structures in programming that allow you to store multiple values of the same type under a single name. They provide a powerful way to manage and manipulate collections of data efficiently. In this blog post, we'll delve into the essentials of arrays in C# and explore various operations and techniques.
Understanding Arrays in C#
Declaring and Initializing Arrays
Arrays in C# are declared using square brackets []
after the data type, followed by the array name and optional initial values.
// Declaring and initializing an array of integers
int[] numbers = new int[] { 1, 2, 3, 4, 5 };
Accessing Array Elements
Individual elements within an array are accessed using zero-based indexing.
int thirdNumber = numbers[2]; // Accesses the third element (index 2) of the array
Modifying Array Elements
You can modify array elements directly by assigning new values to them.
numbers[4] = 10; // Modifies the fifth element (index 4) to have the value 10
Iterating Through Arrays
Looping constructs such as for
and foreach
are commonly used to iterate through array elements.
// Using a for loop to iterate through array elements
for (int i = 0; i < numbers.Length; i++)
{
Console.WriteLine(numbers[i]);
}
// Using foreach loop for more concise iteration
foreach (int num in numbers)
{
Console.WriteLine(num);
}
Multi-dimensional Arrays
C# supports multi-dimensional arrays, including rectangular and jagged arrays.
// Rectangular array (2D)
int[,] matrix = new int[3, 3];
// Jagged array (array of arrays)
int[][] jaggedArray = new int[3][];
jaggedArray[0] = new int[] { 1, 2 };
jaggedArray[1] = new int[] { 3, 4, 5 };
jaggedArray[2] = new int[] { 6, 7, 8, 9 };
Practical Applications of Arrays
Arrays are widely used in various scenarios, including:
- Storing and processing collections of data efficiently.
- Implementing algorithms such as sorting and searching.
- Representing matrices and grids in mathematical computations.
Best Practices for Using Arrays
- Initialization: Always initialize arrays with appropriate sizes and values.
- Bounds Checking: Ensure to check array bounds to avoid accessing out-of-range elements.
- Memory Management: Be mindful of memory usage, especially with large arrays.
Conclusion
Arrays are versatile and indispensable tools in C# programming, offering efficient data storage and manipulation capabilities. By mastering arrays and understanding their nuances, developers can build robust and scalable applications. This blog post has provided a solid foundation in arrays, from basic operations to advanced techniques, empowering you to leverage arrays effectively in your projects.
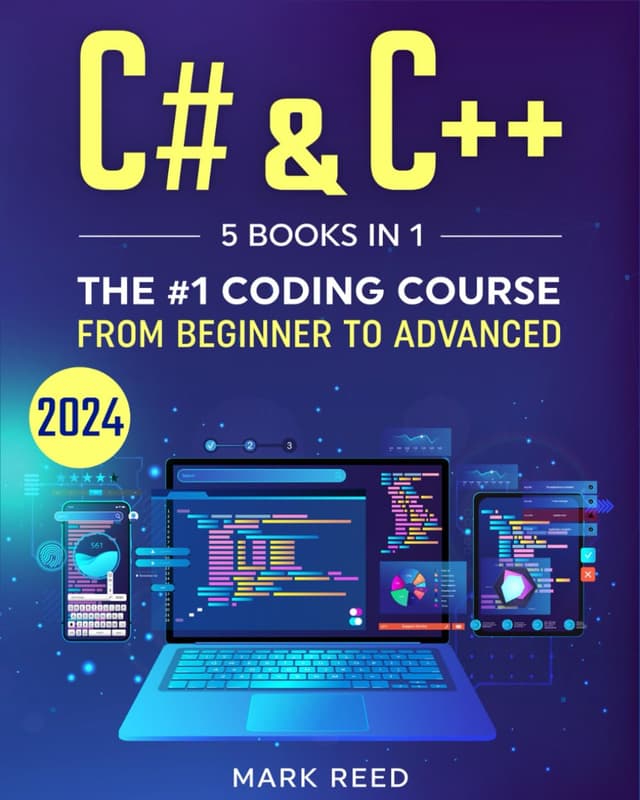