Introduction
Lists in Python are one of the most versatile and widely-used data structures in computer science. They allow you to store and manipulate a collection of items, providing a range of functionalities to handle data efficiently. In this article, we will delve into the concept of lists in Python, exploring their creation, modification, and various methods with practical examples. We will also discuss list comprehension and how lists differ from arrays.
What is a List?
A list in Python is an ordered collection of items, which can be of different data types. Lists are mutable, meaning you can modify their content after creation. This flexibility makes lists a powerful tool for managing data in your programs.
Creating a List
You can create a list by placing items inside square brackets []
, separated by commas. Here's an example:
# Creating a list of integers
numbers = [1, 2, 3, 4, 5]
# Creating a list of strings
fruits = ['apple', 'banana', 'cherry']
# Creating a mixed list
mixed_list = [1, 'apple', 3.5, True]
List Comprehension
List comprehension provides a concise way to create lists. It is a syntactic construct that allows you to generate a list based on an existing list or range.
# Creating a list of squares using list comprehension
squares = [x**2 for x in range(1, 6)]
print(squares) # Output: [1, 4, 9, 16, 25]
Common List Methods
Python lists come with a variety of built-in methods that make data manipulation straightforward. Here are some commonly used methods:
- append(): Adds an item to the end of the list.
- length(): Returns the number of items in the list using
len()
. - pop(): Removes and returns the item at the specified index (default is the last item).
- to string: Converts the list to a string representation.
- sort(): Sorts the items of the list in ascending order.
- remove(): Removes the first occurrence of the specified item.
Let's look at examples of these methods:
# Initializing a list
numbers = [4, 2, 8, 6, 10]
# Appending an item
numbers.append(12)
print(numbers) # Output: [4, 2, 8, 6, 10, 12]
# Getting the length of the list
length = len(numbers)
print(length) # Output: 6
# Popping an item
popped_item = numbers.pop()
print(popped_item) # Output: 12
print(numbers) # Output: [4, 2, 8, 6, 10]
# Sorting the list
numbers.sort()
print(numbers) # Output: [2, 4, 6, 8, 10]
# Removing an item
numbers.remove(4)
print(numbers) # Output: [2, 6, 8, 10]
# Converting list to string
list_string = ', '.join(map(str, numbers))
print(list_string) # Output: "2, 6, 8, 10"
Working with Files in Directory
You can use lists to work with files in a directory. For instance, you can list all files in a directory using the os
module and store them in a list.
import os
# Listing all files in the current directory
files = os.listdir('.')
print(files)
Conclusion
Lists are a fundamental data structure in Python and computer science, offering flexibility and a wide range of functionalities. Understanding how to create, modify, and utilize lists with various methods, including list comprehension, is essential for efficient data management. By mastering lists, you can enhance your ability to write dynamic and robust Python programs.
In summary, lists in Python are powerful tools that allow for comprehensive data manipulation. Whether you're working with files in a directory or performing complex data operations, lists provide the necessary methods to achieve your goals effectively.
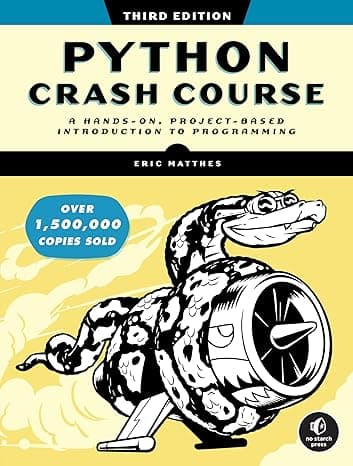