Introduction
Unit testing is a fundamental practice in software development where individual components, or units, of a program are tested to verify their correctness. This article explores unit testing in C#, its benefits, and provides practical examples using a simple Calculator
class to illustrate its application.
What is Unit Testing?
Unit testing involves writing automated tests to validate the behavior of each unit, typically a method or function, in isolation from the rest of the codebase. It helps identify bugs early in the development process and ensures that each unit functions correctly according to its specifications.
Getting Started with Unit Testing in C#
In C#, unit tests are commonly written using frameworks like MSTest, NUnit, or xUnit. These frameworks provide tools to define tests, execute them automatically, and assert expected outcomes.
Example: Testing a Simple Calculator Class
Let’s explore unit testing with a basic Calculator
class that performs addition and subtraction:
using Microsoft.VisualStudio.TestTools.UnitTesting;
public class Calculator
{
public int Add(int a, int b)
{
return a + b;
}
public int Subtract(int a, int b)
{
return a - b;
}
}
[TestClass]
public class CalculatorTests
{
[TestMethod]
public void Add_ShouldReturnCorrectSum()
{
Calculator calc = new Calculator();
int result = calc.Add(3, 5);
Assert.AreEqual(8, result);
}
[TestMethod]
public void Subtract_ShouldReturnCorrectDifference()
{
Calculator calc = new Calculator();
int result = calc.Subtract(10, 4);
Assert.AreEqual(6, result);
}
}
Understanding the Example
In this example:
- Calculator Class: Defines
Add
andSubtract
methods for performing arithmetic operations. - CalculatorTests Class: Contains unit tests using MSTest framework to verify the behavior of
Add
andSubtract
methods:- Add Method Test: Verifies that
Add(3, 5)
returns 8. - Subtract Method Test: Verifies that
Subtract(10, 4)
returns 6.
- Add Method Test: Verifies that
Benefits of Unit Testing
- Early Bug Detection: Identifies issues early in development, preventing them from propagating to later stages.
- Enhanced Code Quality: Ensures that each unit of code behaves as intended, leading to more reliable and maintainable software.
- Facilitates Refactoring: Allows developers to modify code confidently, knowing that existing functionality is validated by tests.
Conclusion
Unit testing is essential for verifying the correctness of individual software components. By adopting unit testing practices in C#, developers can improve code reliability, accelerate development cycles, and build more robust applications.
By integrating unit tests into the development workflow, teams can achieve higher code quality, reduce bugs, and deliver software that meets user expectations consistently.
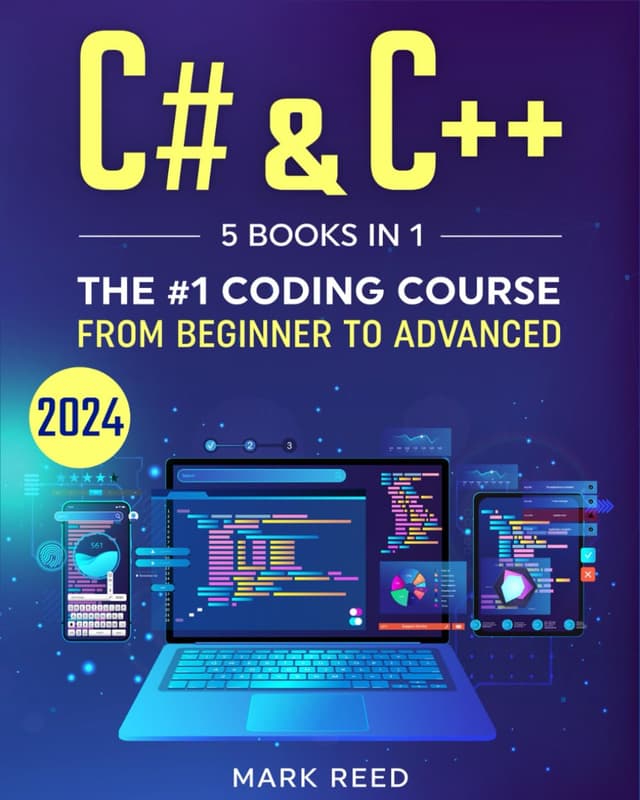