Introduction
In computer science, the if statement is a fundamental control structure that allows programs to make decisions based on conditions. It evaluates a condition and executes a block of code if the condition is true. This concept is essential for creating dynamic and responsive applications. In this article, we will explore the if statement in Python, its syntax, usage, and practical examples.
Understanding the If Statement
The if statement is used to test a condition and execute code only if that condition is true. If the condition is false, the code block is skipped. This enables programmers to direct the flow of the program based on varying conditions.
Basic Syntax
Here’s the basic syntax of an if statement in Python:
if condition:
# Code to execute if the condition is true
Example: Simple If Statement
Let's start with a simple example that demonstrates the use of an if statement to check a condition.
number = 10
if number > 5:
print("The number is greater than 5.")
In this example, the condition number > 5
is true, so the program outputs "The number is greater than 5."
Using Else Clause
Often, you’ll need to execute an alternative block of code if the condition is false. This is where the else clause comes in handy.
number = 3
if number > 5:
print("The number is greater than 5.")
else:
print("The number is not greater than 5.")
In this example, the condition number > 5
is false, so the program outputs "The number is not greater than 5."
Using Elif Clause
When you need to check multiple conditions, you can use the elif clause to test additional conditions.
number = 7
if number > 10:
print("The number is greater than 10.")
elif number > 5:
print("The number is greater than 5 but less than or equal to 10.")
else:
print("The number is 5 or less.")
Here, the program evaluates multiple conditions in sequence. Since number > 5
is true, it outputs "The number is greater than 5 but less than or equal to 10."
Multiple Conditions with and
and or
You can combine conditions using and
and or
operators:
a = 5
b = 10
c = 3
# Example with 'and' condition
if a > 3 and c > 2:
print("Both conditions are true.")
# Example with 'or' condition
if a > 8 or b > 15:
print("At least one condition is true.")
In the first example, the program checks if both a > 3
and c > 2
are true, and prints "Both conditions are true" if they are. In the second example, it checks if either a > 8
or b > 15
is true, and prints "At least one condition is true" if either condition holds.
One-Line If Statement
You can condense an if statement into one line for simple cases:
number = 6
print("The number is greater than 5.") if number > 5 else print("The number is 5 or less.")
This one-line if statement checks if number > 5
and prints the appropriate message.
Nested If Statements
You can also nest if statements within each other to handle more complex scenarios.
number = 15
if number > 10:
print("The number is greater than 10.")
if number > 20:
print("The number is also greater than 20.")
else:
print("But the number is 20 or less.")
In this example, since number > 10
is true, the program enters the first if block and outputs "The number is greater than 10." It then checks if number > 20
. Since this condition is false, it outputs "But the number is 20 or less."
Practical Application: Grading System
Let’s apply the if statement to a practical scenario, such as a grading system.
score = 85
if score >= 90:
print("Grade: A")
elif score >= 80:
print("Grade: B")
elif score >= 70:
print("Grade: C")
elif score >= 60:
print("Grade: D")
else:
print("Grade: F")
This program assigns a grade based on the score. Since the score is 85, it outputs "Grade: B."
Conclusion
The if statement is a vital control structure in Python that enables decision-making in your programs. By understanding and using if, elif, and else clauses, you can create flexible and responsive applications that adapt to various conditions. Mastering the if statement will significantly enhance your ability to write dynamic and efficient code.
In summary, the if statement is an indispensable tool in Python programming, providing the capability to control the flow of your application based on dynamic conditions.
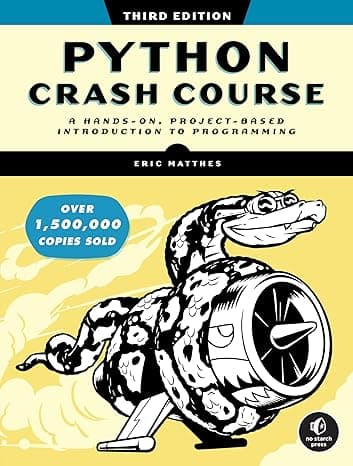