Introduction
In computer science, loops are essential for executing a block of code multiple times. One of the most commonly used loops is the for loop. It provides a concise way to iterate over a sequence of values, making it a powerful tool for repetitive tasks. In this article, we’ll explore the for loop in C#, covering its syntax, usage, and practical examples to help you understand how to use it effectively in your programs.
Understanding the For Loop
A for loop is a control structure that allows you to repeat a block of code a specific number of times. It is particularly useful when you know in advance how many times you want to execute a statement or a block of statements.
Basic Syntax
Here’s the basic syntax of a for loop in C#:
for (initialization; condition; iteration)
{
// Code to be executed
}
- Initialization: Sets up the loop variable and is executed once at the start of the loop.
- Condition: Evaluated before each iteration; if true, the loop continues. If false, the loop terminates.
- Iteration: Executes after each iteration of the loop body, usually used to update the loop variable
Example: Simple For Loop
Let's start with a simple example that demonstrates the use of a for loop to print numbers from 1 to 10.
class Program
{
static void Main()
{
for (int i = 1; i <= 10; i++)
{
Console.WriteLine(i);
}
}
}
In this example, the loop initializes i
to 1, continues as long as i
is less than or equal to 10, and increments i
by 1 after each iteration, printing the numbers 1 through 10.
Using For Loops with Arrays
For loops are often used to iterate over arrays or collections. Here’s an example of using a for loop to iterate through an array of integers.
class Program
{
static void Main()
{
int[] numbers = { 1, 2, 3, 4, 5 };
for (int i = 0; i < numbers.Length; i++)
{
Console.WriteLine(numbers[i]);
}
}
}
This loop iterates over each element in the numbers
array, printing each value.
Nested For Loops
For loops can be nested within other for loops to handle more complex scenarios, such as printing a multiplication table.
class Program
{
static void Main()
{
for (int i = 1; i <= 10; i++)
{
for (int j = 1; j <= 10; j++)
{
Console.Write($"{i * j}\t");
}
Console.WriteLine();
}
}
}
In this example, the outer loop iterates through the numbers 1 to 10, and the inner loop does the same. The product of i
and j
is printed, resulting in a multiplication table.
Practical Application: Summing Array Elements
Let’s use a for loop to calculate the sum of elements in an array.
class Program
{
static void Main()
{
int[] numbers = { 10, 20, 30, 40, 50 };
int sum = 0;
for (int i = 0; i < numbers.Length; i++)
{
sum += numbers[i];
}
Console.WriteLine($"The sum of the array elements is: {sum}");
}
}
This program initializes the sum to 0, then iterates over each element in the numbers
array, adding each element to sum
. Finally, it prints the total sum.
Benefits of Using For Loops
- Clarity and Readability: For loops provide a clear and concise way to iterate over a sequence of values, making the code easier to read and understand.
- Efficiency: For loops are generally more efficient than other loop constructs when the number of iterations is known beforehand.
- Control: The for loop offers precise control over the loop variable, condition, and iteration, allowing for more complex looping scenarios.
Conclusion
The for loop is a fundamental control structure in C# that enables repetitive execution of code blocks. By mastering the for loop, you can handle a wide range of programming tasks more efficiently and effectively. Understanding its syntax and practical applications will significantly enhance your ability to write robust and efficient code.
In summary, the for loop is an essential tool in C# programming, providing a structured and efficient way to handle repetitive tasks and iterate over collections.
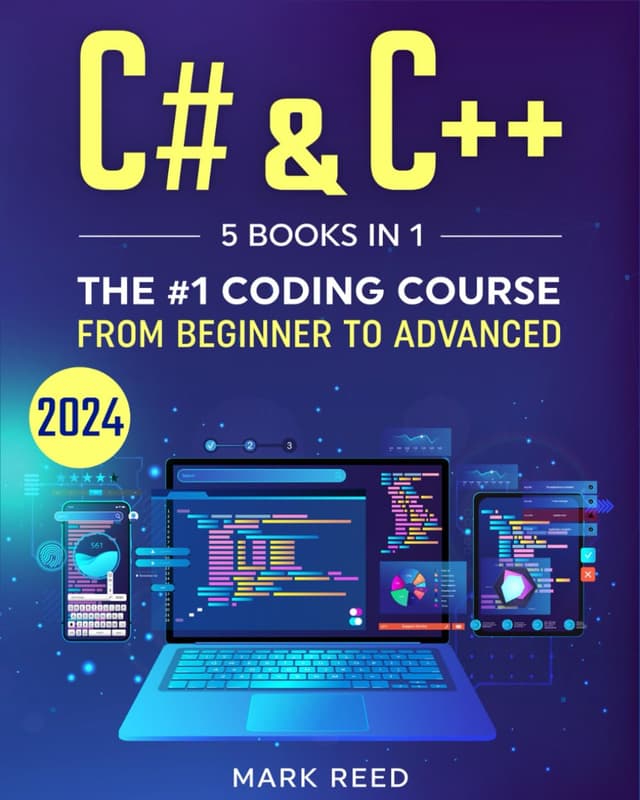