Introduction
Encapsulation is a fundamental concept in object-oriented programming (OOP) that promotes the bundling of data (attributes) and methods (behaviors) that operate on the data into a single unit known as a class. This article explores encapsulation in C#, its principles, benefits, and practical examples to demonstrate its importance in software development.
What is Encapsulation?
In computer science, encapsulation refers to the bundling of data (attributes or fields) and methods (functions or procedures) that manipulate the data into a single unit. This unit is called a class, where the data is hidden from the outside world and can only be accessed through public methods. Encapsulation helps in achieving data hiding, abstraction, and modularity in code, promoting better organization and security.
Principles of Encapsulation
- Data Hiding: Encapsulation hides the internal state (data) of an object from outside interference or misuse. The internal state can only be modified by the methods provided by the class, ensuring data integrity and security.
- Abstraction: Encapsulation allows for abstraction, where the complex internal details of how data is stored and manipulated are hidden behind a simple interface. Users of the class only need to know how to use its public methods, not how they are implemented.
- Access Control: Encapsulation provides control over how data can be accessed and modified. By using access modifiers (like public, private, protected), developers can specify the visibility and accessibility of data members and methods.
Example: Encapsulation in C#
Let's illustrate encapsulation with a simple example in C#:
public class Car
{
private string model;
private int year;
public string Model
{
get { return model; }
set { model = value; }
}
public int Year
{
get { return year; }
set { year = value; }
}
public void DisplayDetails()
{
Console.WriteLine($"Model: {model}, Year: {year}");
}
}
class Program
{
static void Main()
{
Car myCar = new Car();
myCar.Model = "Toyota Camry";
myCar.Year = 2022;
myCar.DisplayDetails();
}
}
In this example:
- The
Car
class encapsulates themodel
andyear
fields, which are private and can only be accessed or modified through the public propertiesModel
andYear
. - The
DisplayDetails()
method provides a controlled way to display the car's details, encapsulating the implementation details from the outside world.
Benefits of Encapsulation
- Modularity: Encapsulation promotes modularity by dividing complex systems into smaller, manageable classes. Each class encapsulates its own data and behaviors, making the code more organized and easier to maintain.
- Security: By hiding internal data and exposing only necessary methods, encapsulation prevents unauthorized access and manipulation of data, enhancing security.
- Code Reusability: Encapsulated classes can be reused in different parts of a program or in other programs without modification, promoting code reusability and reducing redundancy.
Conclusion
Encapsulation is a foundational principle in object-oriented programming, crucial for building maintainable, secure, and reusable software systems. By encapsulating data and methods within classes, developers can achieve better organization, security, and modularity in their code, ultimately leading to more robust and scalable applications.
In summary, understanding and applying encapsulation in C# empowers developers to write cleaner, more efficient code that is easier to maintain and extend.
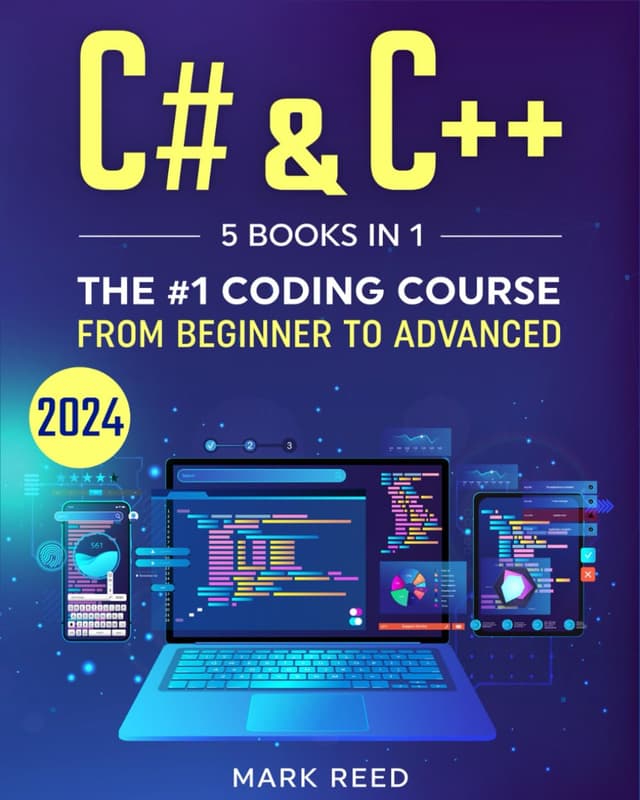