Introduction to class
In object-oriented programming (OOP), classes serve as the fundamental building blocks that enable developers to create reusable and organized code. C# (pronounced as C-sharp), a versatile programming language developed by Microsoft, utilizes classes extensively to structure applications. This article dives deep into the concept of classes in C#, exploring their significance, structure, and practical implementation.
Object-Oriented Programming in C#
What is a Class?
At its core, a class in C# can be thought of as a blueprint or template for creating objects. It defines the data (attributes or properties) and behaviors (methods or functions) that objects of the class will exhibit. This concept is pivotal in OOP as it promotes modularity, reusability, and maintainability of code.
Anatomy of a Class
Let’s dissect the structure of a class in C#:
// Example of a simple class in C#
public class Car
{
// Fields or properties
public string Model { get; set; }
public string Manufacturer { get; set; }
public int Year { get; set; }
// Constructor
public Car(string model, string manufacturer, int year)
{
Model = model;
Manufacturer = manufacturer;
Year = year;
}
// Method
public void Start()
{
Console.WriteLine($"Starting the {Year} {Manufacturer} {Model}...");
// Additional logic to start the car
}
}
Key Components:
- Fields or Properties: These are the attributes of the class. In the example above,
Model
,Manufacturer
, andYear
are properties of theCar
class. - Constructor: This special method is invoked when an object of the class is instantiated. It initializes the object’s state. In our
Car
class, the constructor initializes the propertiesModel
,Manufacturer
, andYear
. - Methods: These are functions defined within the class that define its behavior. The
Start
method in ourCar
class simulates starting the car.
Instantiating Objects
Once a class is defined, objects (instances) can be created from it:
class Program
{
static void Main()
{
// Creating an object of the Car class
Car myCar = new Car("Civic", "Honda", 2023);
// Accessing properties
Console.WriteLine($"My car is a {myCar.Year} {myCar.Manufacturer} {myCar.Model}");
// Calling methods
myCar.Start();
}
}
Benefits of Using Classes
- Code Organization: Classes promote a structured approach to programming, making code easier to manage and understand.
- Code Reusability: Once a class is defined, it can be instantiated multiple times, promoting code reusability across different parts of an application.
- Encapsulation: Classes encapsulate data (properties) and behaviors (methods), hiding the internal implementation details from the outside world.
Conclusion
Understanding classes in C# is foundational to becoming proficient in object-oriented programming. By defining classes, developers can create modular, reusable code that enhances the scalability and maintainability of their applications. As you delve deeper into C# programming, mastering classes will empower you to build robust software solutions efficiently.
In summary, classes in C# are powerful tools that facilitate the creation of organized and efficient code structures, contributing significantly to the principles of object-oriented programming.
Related Content:
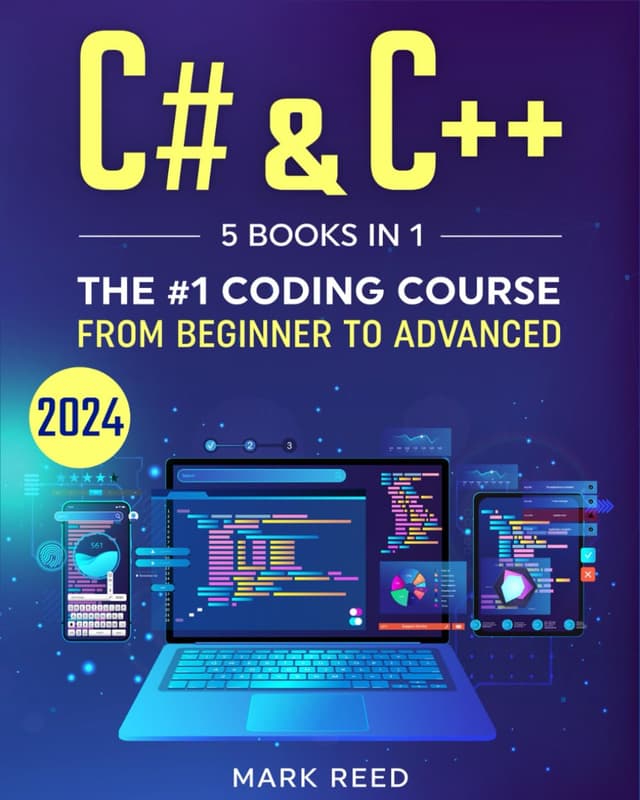