Introduction
Arrays are a fundamental concept in computer science and programming. They provide a way to store multiple values in a single variable, making it easier to manage and manipulate data. In this blog post, we will explore the concept of arrays, understand their importance, and see how they can be used effectively.
What is an Array?
An array is a collection of items stored at contiguous memory locations. The idea is to store multiple items of the same type together. This makes it easier to calculate the position of each element by simply adding an offset to a base value, such as the memory location of the first element of the array (typically denoted by the name of the array).
Why Use Arrays?
Arrays are used for various reasons:
- Efficiency: Accessing elements by index is fast.
- Organization: Helps in organizing data in a structured way.
- Memory Management: Allows managing data efficiently in memory.
- Easy Manipulation: Provides various methods to add, remove, and modify elements.
Practical Example: Simple Student Grades System
Let's create a simple program that stores student grades and calculates the average grade.
# Importing array module
import array
# Create an array of student grades
grades = array.array('i', [85, 90, 78, 92, 88])
# Calculate the average grade
average_grade = sum(grades) / len(grades)
print(f"Average Grade: {average_grade}")
# Find the highest and lowest grades
highest_grade = max(grades)
lowest_grade = min(grades)
print(f"Highest Grade: {highest_grade}")
print(f"Lowest Grade: {lowest_grade}")
Conclusion
Arrays are a vital part of computer science and programming. They provide a structured way to store and manage data efficiently. By understanding how to create, access, modify, and manipulate arrays, you can efficiently manage collections of data in your programs. Practice using arrays in various scenarios to get a strong grasp of this essential concept.
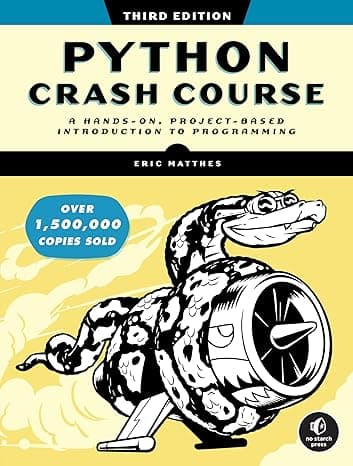